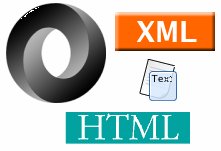
Some time ago, Peter Paul Kosh wrote an article about the different types of Ajax data formats - The AJAX response: XML, HTML, or JSON?. I know that it is a bit late but this is my views on the topic.
JSON
I prefer JSON as it is the easiest to parse - just put an 'eval' and we are done. My biggest issue with this is the text created by the server-side script is next to unreadable by human beings. This makes it very hard to debug our Ajax scripts.
Text
My second favorite place goes to Plain text format as it is the easiest to generate. I use plain text only when there is just a single unit of data - when I want the email address of an user from the database or if I want to know wether the given username exists in the database - the response data will be just a '1' or a '0' in this case.
AHAH
Next in line comes the HTML format - the easist to display. $("display").innerHTML = http.responseText
is all it takes to get the job done. This method is commenly known as AHAH.
XML
Last but not the least comes the XML format - the most portable solution. Unfortunatly, XML format is the most difficult to parse.
My jx Ajax Library provides support for both Text and JSON data formats. One can use the Text support of this library for getting HTML data. It does not support XML format as of yet and I have no intension to add that support to my library as I want to keep it as small as possible.
So I created another small Ajax with XML library called xjx that would do the trick...
///////////////////////////////////// xjx - XML Ajax Library //////////////////////////////////////
var xjx = {
"xml_file":false,
"xml":false,
"callback":false,
"handler":function () {//Firefox only function - happens when a XML file is loaded
if(!this) {
xjx.error("Standard");
return false;
}
if(xjx.callback) xjx.callback(this);
},
//Load the xml file - using different method for different browsers
"load":function (xml_file,callback) {
this.file = xml_file;
this.callback = callback;
var xmlDocument;
if(document.implementation.createDocument) {//Firefox
xmlDocument = document.implementation.createDocument('', '', null);
xmlDocument.load(xml_file);
xmlDocument.addEventListener('load', this.handler, false); //This function will happen when the file is loaded
}
else { //IE
xmlDocument = new ActiveXObject('Microsoft.XMLDOM');
xmlDocument.async = false;
var loadResult = xmlDocument.load(xml_file);
if (loadResult) {
if(callback) callback(xmlDocument);
}
else {
this.error("ActiveX");
return false;
}
}
this.xml = xmlDocument;
return true;
},
"error":function (from) {//Any error messages?
alert("Error reading the XML file '" + this.xml_file + "'");
return false;
}
};
You can call it from your script like this...
xjx.load("file.xml",function(xmlDoc) {
processXMLDoc(xmlDoc);
});
0 Comments:
Post a Comment